KoreanFoodie's Study
SW 역량테스트 - [모의 SW 역량테스트] 줄기세포배양 문제 풀이/해답/코드 (C++ / JAVA) 본문
Data Structures, Algorithm/SW 역량테스트
SW 역량테스트 - [모의 SW 역량테스트] 줄기세포배양 문제 풀이/해답/코드 (C++ / JAVA)
GoldGiver 2020. 9. 29. 11:47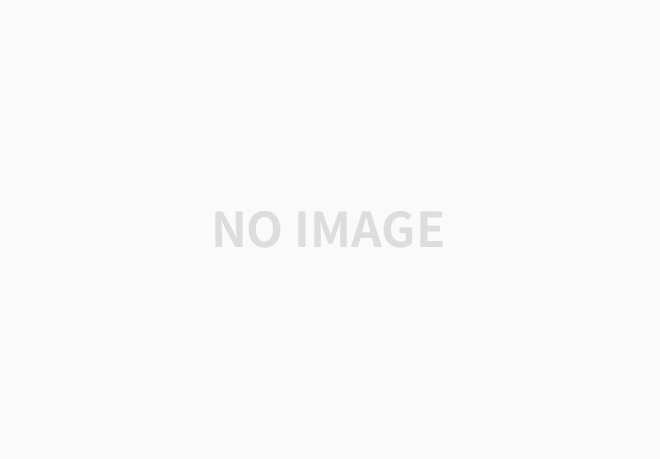
SW 역량 테스트 준비를 위한 핵심 문제들을 다룹니다!
해답을 보기 전에 문제를 풀어보시거나, 설계를 하고 오시는 것을 추천드립니다.
코드에 대한 설명은 주석을 참고해 주세요 :)
문제 링크 : swexpertacademy.com/main/code/problem/problemDetail.do?contestProbId=AWXRJ8EKe48DFAUo
해답 코드 :
#include <iostream>
#include <vector>
#include <algorithm>
using namespace std;
typedef struct cell {
int row;
int col;
int deact;
int act;
int X;
}cell ;
typedef struct info {
int alloc;
int X;
}info;
int M, N, K;
int dR[4] = {-1, 0, 1, 0};
int dC[4] = {0, 1, 0, -1};
info map[360][360];
bool dead(cell c) {
if (c.deact == 0 && c.act == 0) return true;
else return false;
}
int main(int argc, char** argv)
{
int test_case;
int T;
/*
아래의 freopen 함수는 input.txt 를 read only 형식으로 연 후,
앞으로 표준 입력(키보드) 대신 input.txt 파일로부터 읽어오겠다는 의미의 코드입니다.
//여러분이 작성한 코드를 테스트 할 때, 편의를 위해서 input.txt에 입력을 저장한 후,
freopen 함수를 이용하면 이후 cin 을 수행할 때 표준 입력 대신 파일로부터 입력을 받아올 수 있습니다.
따라서 테스트를 수행할 때에는 아래 주석을 지우고 이 함수를 사용하셔도 좋습니다.
freopen 함수를 사용하기 위해서는 #include <cstdio>, 혹은 #include <stdio.h> 가 필요합니다.
단, 채점을 위해 코드를 제출하실 때에는 반드시 freopen 함수를 지우거나 주석 처리 하셔야 합니다.
*/
//FILE *fp;
//freopen_s(&fp, "sample_input.txt", "r", stdin);
cin >> T;
/*
여러 개의 테스트 케이스가 주어지므로, 각각을 처리합니다.
*/
for (test_case = 1; test_case <= T; ++test_case)
{
// 0 : empty, not added
// 1 : not empty, newly added
// 2 : not empty, old (or dead)
vector<cell> myL;
cin >> N >> M >> K;
int new_i = (360 / 2) - (N/2);
int new_j = (360 / 2) - (M/2);
int input_t;
cell input_q;
for (int i = 0; i < N; i++) {
for (int j = 0; j < M; j++) {
cin >> input_t;
if (input_t > 0) {
input_q = { new_i + i, new_j + j, input_t, input_t, input_t };
myL.push_back(input_q);
}
}
}
// copy information from list to map
for (int i = 0; i < myL.size(); i++) {
map[myL[i].row][myL[i].col].alloc = 2;
map[myL[i].row][myL[i].col].X = myL[i].X;
}
int n_row;
int n_col;
cell t_cell;
// temporarily added cells
vector<cell> newL;
// update for K-loop
for (int tick = 0; tick < K; tick++) {
// traverse queue, fill empty cells
for (int i = 0; i < myL.size(); i++) {
t_cell = myL[i];
if (t_cell.deact > 0) {
continue;
} // spread it
else if (t_cell.act > 0) {
for (int dir = 0; dir < 4; dir++) {
n_row = t_cell.row + dR[dir];
n_col = t_cell.col + dC[dir];
// totally empty
if (map[n_row][n_col].alloc == 0) {
map[n_row][n_col].alloc = 1;
map[n_row][n_col].X = t_cell.X;
cell new_cell = {n_row, n_col, t_cell.X, t_cell.X, t_cell.X};
newL.push_back(new_cell);
} // not empty, but newly added
else if (map[n_row][n_col].alloc == 1) {
// if smaller, pass
if (map[n_row][n_col].X >= t_cell.X) {
continue;
} // if larger, update the X value
else {
map[n_row][n_col].X = t_cell.X;
for (int j = 0; j < newL.size(); j++) {
if (newL[i].row == n_row && newL[i].col == n_col) {
newL[i].X = t_cell.X;
}
}
}
}
}
}
}
// clock is ticking -> update time
for (int i = 0; i < myL.size(); i++) {
if (myL[i].deact > 0) {
myL[i].deact--;
}
else {
myL[i].act--;
}
}
// update information of myQ into the map -> make all old
for (int i = 0; i < newL.size(); i++) {
map[newL[i].row][newL[i].col].alloc = 2;
}
// remove all dead cells
myL.erase(remove_if(myL.begin(), myL.end(), dead), myL.end());
// merge 2 lists
myL.insert(myL.end(), newL.begin(), newL.end());
// clear newL
newL.clear();
}
// initialize map
info clean = { 0, 0 };
fill(&map[0][0], &map[359][359], clean);
cout << "#" << test_case << " " << myL.size() << endl;
}
return 0;//정상종료시 반드시 0을 리턴해야합니다.
}
SW 역량테스트 준비 - [모의 SW 역량테스트] 풀이 / 코드 / 답안 (C++ / JAVA)
SW 역량테스트 준비 - C++ 코드, Java 코드
SW 역량테스트 준비 - 백준 알고리즘 문제
'Data Structures, Algorithm > SW 역량테스트' 카테고리의 다른 글
SW 역량테스트 - [백준] 구슬탈출2 문제 풀이/해답/코드 (C++ / JAVA) (0) | 2020.09.30 |
---|---|
SW 역량테스트 - [모의 SW 역량테스트] 원자 소멸 시뮬레이션 문제 풀이/해답/코드 (C++ / JAVA) (0) | 2020.09.29 |
SW 역량테스트 - [백준] 톱니바퀴 문제 풀이/해답/코드 (C++ / JAVA) (0) | 2020.09.29 |
SW 역량테스트 - [모의 SW 역량테스트] 미생물 격리 문제 풀이/해답/코드 (C++ / JAVA) (1) | 2020.09.29 |
SW 역량테스트 - [모의 SW 역량테스트] 활주로 건설 문제 풀이/해답/코드 (C++ / JAVA) (0) | 2020.09.29 |
Comments