목록Tutorials/Scala (11)
KoreanFoodie's Study
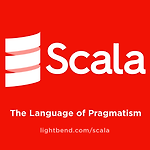
스칼라 튜토리얼, scala의 Abstract class에 대해 알아보자 Abstract Class: Specification Abstract Classes can be used to abstract away the implementation details. In short, abstract classes is used for specfication, and concrete sub-classes for implementation. Example: Abstract case for specification abstract class Iter[A] { def getValue: Option[A] def getNext: Iter[A] } def sumElements[A](f: A=>Int)(xs: Iter[A])..
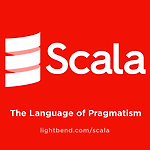
스칼라 튜토리얼, scala의 class에 대해 알아보자 Class: Parameterized Record object gee { val a : Int = 10 def b : Int = a + 20 def f(z: Int) : Int = b + 20 + z } type gee_type = {val a:Int; def b: Int; def f(z:Int): Int} class foo_type(x: Int, y: Int) { val a : Int = x def b : Int = a + y def f(z: Int) : Int = b + y + z } val foo : foo_type = new foo_type(10,20) If you define class, new type is made. The name r..
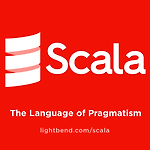
스칼라 튜토리얼, scala의 sub types에 대해 알아보자 Sub Type Polymorphism (Concept) Let's assume that we want this: object tom { val name = "Tom" val home = "02-880-1234" } object bob { val name = "Bob" val home = "02-123-1234" } def greeting(r: ???) = "Hi " + r.name + ", How are you?" greeting(tom) greeting(bob) // Note that we have tom: {val name: String; val home: String} bob: {val name: String; val mobile: ..
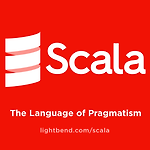
스칼라 튜토리얼, scala의 parametric polymophism에 대해 알아보자 Parametric Polymorphism: Functions Problem def id1(x: Int): Int = x def id2(x: Double): Double = x Can we avoid DRY(Do not Reapeat Yourself)? Parametric Polymorphism(a.k.a. For-all Types) def id[A](x: A): A = x // type for definition val f = (x:Int) => x // type for value The type of id is [A](x: A)A. id is a parametric expression. Only definition..
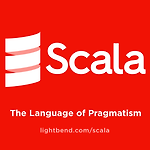
스칼라 튜토리얼, scala datatypes part2 Pattern Matching A way to use algebraic datatypes Syntax: e match { case C1(...) => e1 ... case Cn(...) => en } Example : sealed abstract class IList case class INil() extends IList case class ICons(hd: Int, tl: IList) extends IList def x: IList = ICons(2, ICons(1, INil())) def length(xs: IList) : Int = xs match { case INil() => 0 case ICons(x, tl) => 1 + length(t..
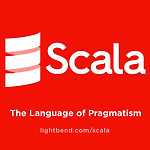
스칼라 튜토리얼, scala의 datatypes에 대해 알아보자 Types in general Introdection : How to construct elements Elimination : How to use elements of the type Primitive types : Int, Boolean, Double, String... Intro for Int: ...,-2,-1,0,1,2 Elim for Int: +,-,*,/... -Function types : Int => Int, (Int => Int)=>(Int => Int) Intro: (x:T)=>e Elim: f(v) Tuples Intro : (1, 2, 3) : (Int, Int, Int) (1, "a") : (Int, String) ..
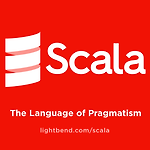
스칼라 튜토리얼, scala에서 currying에 대해 알아보자 Currying Why we use currying? Let's look at the code, simplified by currying. def sum(f: Int=>Int, a: Int, b: Int): Int = if (a Int): (Int, Int) => Int = { def sumF(a: Int, b: Int): Int = if (a Int)(a: Int, b: Int): Int = if (a T can be turned into one of type T1 => (T2 => (... Tn =>T) This is called 'currying' named after Haskell Brooks Curry. The opposite di..
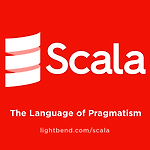
스칼라 튜토리얼, scala의 closure에 대해 알아보자 Parameterized expression vs. values Functions defined using “def” are not values but parameterized expressions. • Anonymous functions are values. • But, parameterized expressions are implicitly converted to values. • Explicit conversion: f _ • Anonymous functions can be seen as syntactic sugar: (x:T)=>e is equivalent to { def \_\_noname(x:T) = e; \_\_noname \_ }..
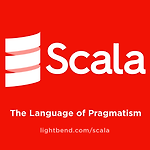
스칼라 튜토리얼, scala의 tail recursion을 알아봅시다 Recursion needs care Let's look at typical summation fuction. def sum(n: Int) : Int = if (n x*x*x, n) // or def sumLinear(n: Int) = sum((x)=>x, n) def sumSquare(n: Int) = sum((x)=>x*x, n) def sumCubes(n: Int) = sum((x)=>x*x*x, n) Excercise def sum(f: Int => Int, a: Int, b: Int): Int = if (a Int, a: Int, b: Int): Int = if (a Int, inival: Int, f: Int => Int, ..
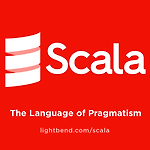
스칼라 튜토리얼, scala에서 lazy val에 대해 알아보자 Blocks and Name Scoping Blocks in scala Block is an expression. It also allow nested name binding. Block allows arbitraty order of "def"s, but not "val"s(think about why). Scope of names Block example : val t = 0 def f(x: Int) = t + g(x) def g(x: Int) = x * x val x = f(5) val r = { val t = 10 val s = f(5) t + s } val y = t + r A definition inside a block is on..